Tesseral Quickstart
Sign up for Tesseral
Go to https://console.tesseral.com and sign up. At a step in the flow, you’ll be asked to Create a Project.
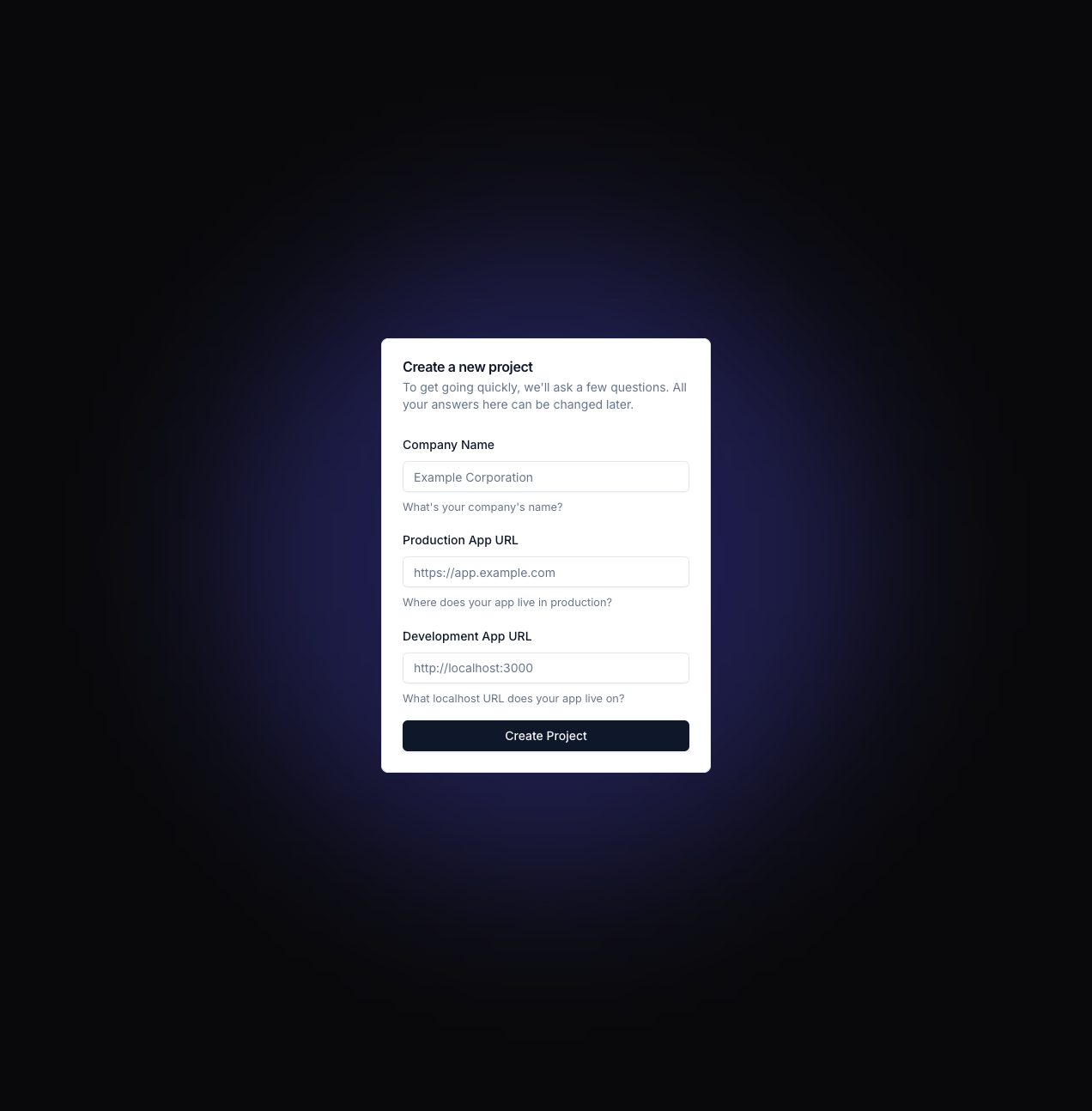
You can change your choices here later. The important thing is:
-
Production App URL is the URL where your production app lives.
Typically, this is something like
https://app.company.com
(sometimesapp.
is instead something likeconsole.
ordashboard.
), but some applications put it onhttps://company.com
. Put whatever style you use; Tesseral supports them all. -
Development App URL is the URL where your app lives when you’re developing on it locally.
Choose something like
http://localhost:3000
. If you have a setup where you run live previews on some kind of preview URL, then you can add those later. Start by adding just your localhost domain.
After you complete this step, Tesseral will automatically create two Tesseral Projects for you: a production Project and a development Project (whose name ends in “Dev”).
You’ll be logged into your development Project. You can switch between your Projects from the Project Switcher at the top left of the Console.
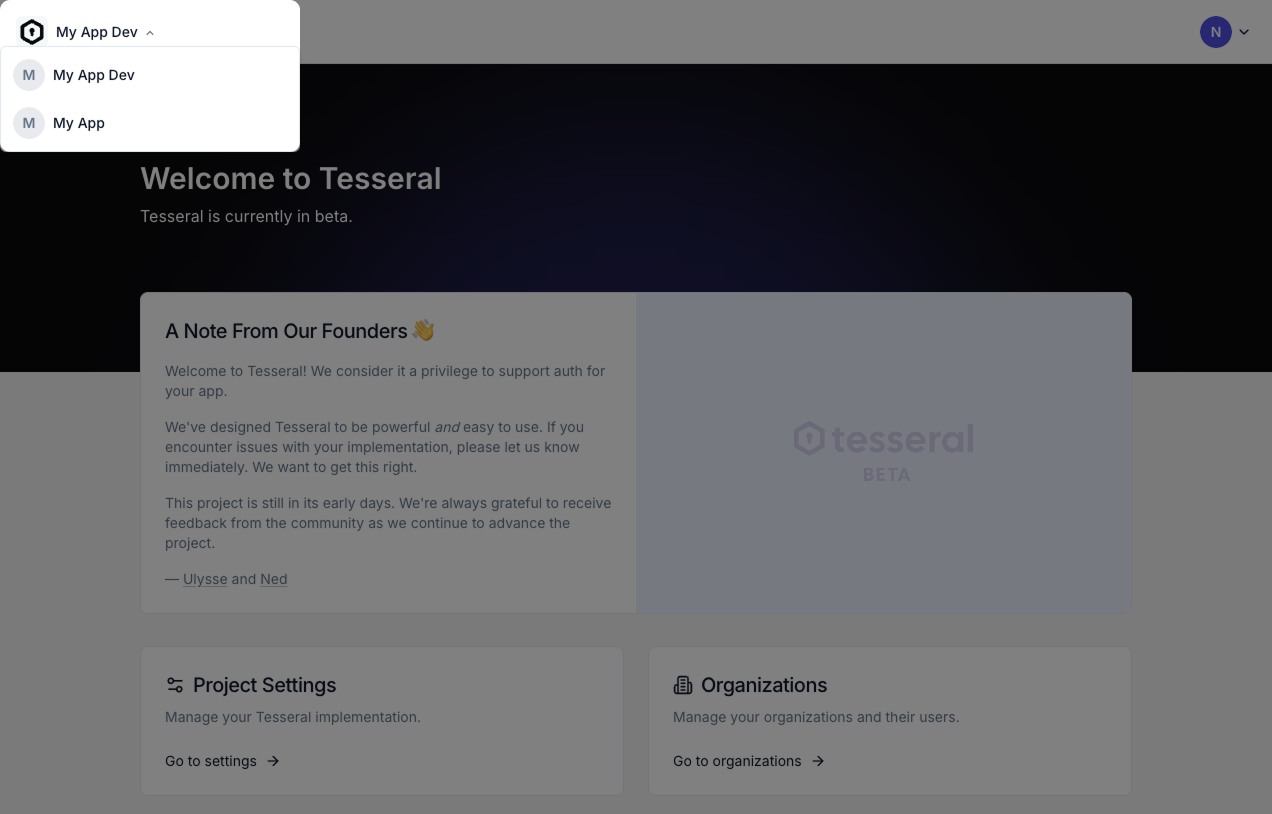
Add Tesseral to your clientside code
Tesseral’s React SDK handles redirecting users to your login page, giving your
React code hooks like useUser()
or useLogout()
, and having your frontend
automatically send authentication information in its requests to your server.
Get your Dev Mode publishable key
Go to https://console.tesseral.com/project-settings/api-keys and click on “Create” under “Publishable Keys”. Make sure to enable “Dev Mode” on the publishable key.
You’ll need the ID of your new publishable key for the next step. It starts with
publishable_key_...
.
Add TesseralProvider
to your app
Find your clientside React root, and add TesseralProvider
there, with the
publishable key ID from the previous step as the publishableKey
prop:
Now, when you visit your webapp, your customers will automatically be redirected to a Tesseral-hosted login flow if they’re not already logged in.
Add Tesseral to your serverside code
Tesseral provides SDKs for Express.js, Flask, and Golang. You’ll need the Publishable Key you created previously.
Express.js
Flask
Go
Install the Tesseral Express.js SDK by running:
And then add requireAuth()
to your Express app:
Replace publishable_key_...
with the same publishable key you used for your
frontend.
All requests to your backend are now server-side authenticated. Anywhere in your
code where you need to know which customer you’re talking to, you can use
organizationId()
:
For more documentation, check out the Tesseral Express.js SDK docs.
Going to Production
In the previous two sections, you added authentication to your frontend and backend. Both of these were running on localhost. To go to production, you need to:
-
Use your production publishable keys, instead of the dev ones you’ve used so far. This is the only code change you need to make.
-
Go into the Tesseral Console, and configure a custom domain for your Project’s Vault. Right now, your production Project uses a Tesseral-assigned URL that looks like
project-[...].tesseral.app
.In production, we recommend you use a domain you control instead of a domain Tesseral provides for you. We recommend you use a domain that looks like
vault.app.company.com
orvault.company.com
, depending on whether you useapp.company.com
orcompany.com
as your App Production URL.
Here is how you configure a custom domain for your Project’s Vault:
-
Go to the Tesseral Console. Click on the Project Switcher at the top left, and click on your production Project (it’s the one whose name doesn’t end in “Dev”).
-
Go to Project Settings, and then the “Vault Domain Settings” tab. Click on “Edit”, and under “Custom Vault Domain” you’ll input
vault.XXX
, whereXXX
is the domain of your App Production URL that you used in “Sign up for Tesseral” above.For example, if you used
https://app.company.com
, then usevault.app.company.com
as your production Project’s Vault Domain.If instead you used
https://company.com
(noapp.
orconsole.
ordashboard.
-like subdomain), then usevault.company.com
as your production Project’s Vault Domain. -
You’ll now get a set of DNS records you need to set up. Create those DNS records. Once those records are all correct and widely propagated, you can enable your Custom Vault Domain.
Now, when your customers are redirected to their login page, they’ll see
vault.app.company.com
. You can also Customize the look here
to make the experience seamless with your company’s brand.