Managed API Keys in Tesseral
Tesseral can make your existing endpoints become a customer-accessible API
Tesseral can manage API keys for your product. With a single line of code, you can opt into making endpoints that your customers can securely access over cURL or HTTP.
What are managed API Keys?
Tesseral not only provides secure authentication for Users, but it also supports secure API key authentication. This allows your customers to call your endpoints using managed API keys instead of user credentials. User credentials only work from a individual’s web browser, but API keys work with cURL or any other program.
When you enable this feature, Tesseral handles the generation of secure secret tokens with 256-bit entropy. These tokens serve as authentication credentials that your customers can safely use to access your API. Tesseral provides SDKs that you can use to verify the authenticity of these secret tokens.
You have fine-grained control over where managed API keys are used. You can enable them only on specific endpoints that you want to expose, maintaining tight control over your API surface.
Managed API keys are fully integrated with Tesseral’s managed role-based access control (RBAC) feature. Your customers can generate keys with scoped permissions, ensuring that each key only has access to the resources it needs.
Enabling API key support on an endpoint typically requires no code changes to that endpoint’s code. You always have control over whether your code is accessible to Users, API Keys, or both.
Enabling Managed API Keys
To get started, you simply toggle the feature on at the project level. You can also selectively enable it for individual organizations—allowing you to control access or monetize it as needed.
Configuring an API Key Prefix
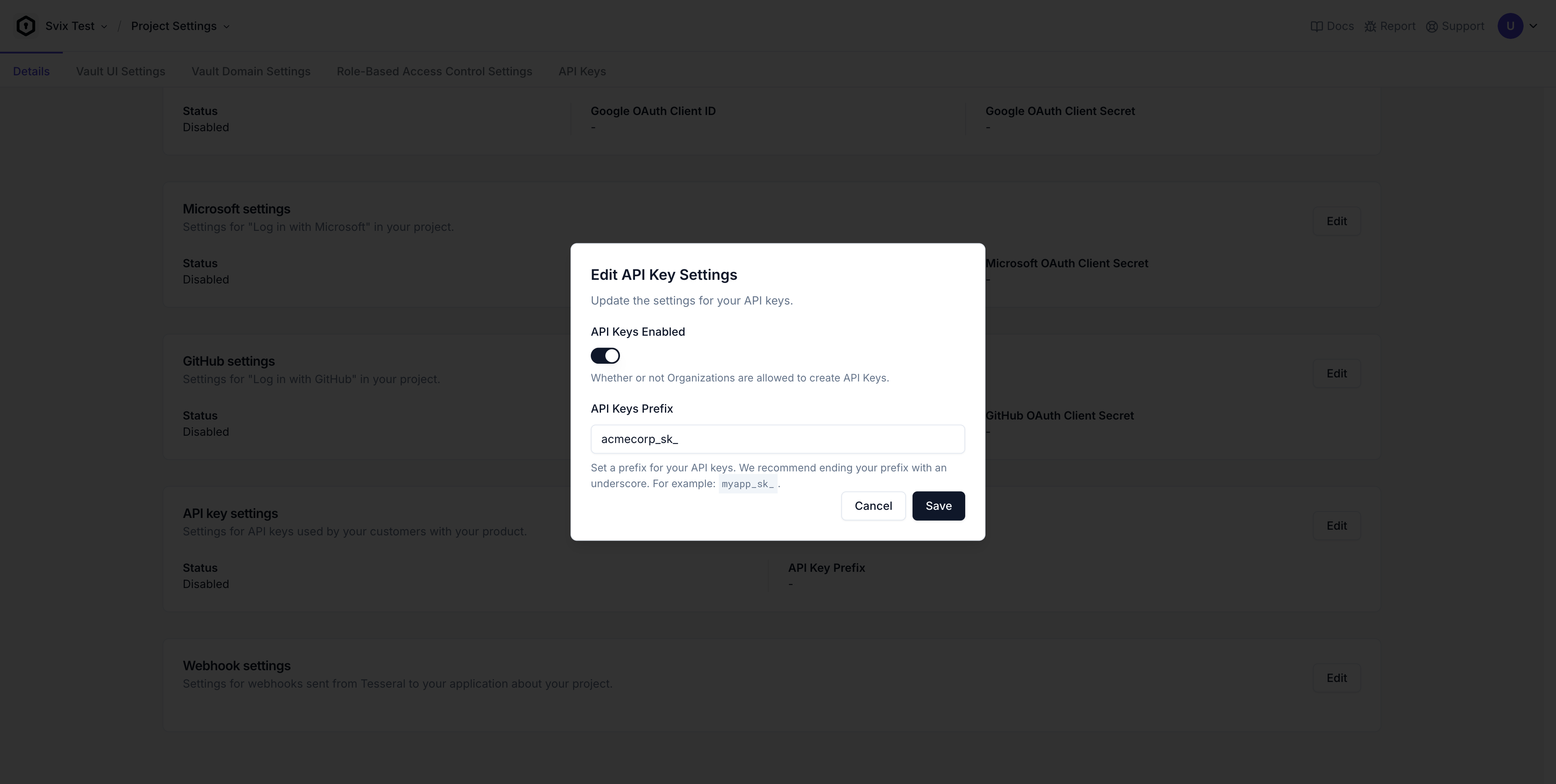
Following a convention established by Stripe, Tesseral’s managed API Keys are formatted as a long alphanumeric string with a prefix of your choosing. You can configure this API Key Prefix from your Project Settings in the Tesseral Console.
For example, if your company is named ACMECorp, you might choose an API Key
prefix of acmecorp_sk_
. Then your API Key secret tokens will look something
like:
Enabling API Keys for an Organization
By default, Organizations in your Project cannot create API Keys. You must enable API Keys on an Organization to add this functionality.
API Keys are not enabled by default because you may want to charge your customers for access to this functionality.
Get a Tesseral Backend API Key
Managed API Keys require use of the Tesseral Backend API under the hood. Tesseral’s serverside SDKs will manage doing those calls for you, but you will need a Tesseral Backend API Key to proceed.
You can create a Tesseral Backend API Key by following these steps:
-
Sign in to the Tesseral Console.
-
Make sure you’re in the Project you want to work with. You can switch between Projects using the Project Switcher at the top left of the Console. 3. Go to your Project’s API Keys Settings, and create a new Backend API Key.
-
When your Project API Key is created, you’ll be given a chance to copy your Project API Key Secret Token. Keep this secret somewhere safe; it is sensitive.
You now have a Backend API Key Secret Token, which starts with:
Put this value in an environment variable called TESSERAL_BACKEND_API_KEY
.
Code Changes
API Keys are a serverside-only concept. You don’t need to change your clientside code at all to support API Keys. When you enable API Keys on an Organization, your customers can view, create, revoke, and delete their API Keys from their Self-Serve Organization Settings.
Your serverside code will not support API Keys by default. To enable support for API Keys, make a one-line change to your code:
Express.js
Flask
FastAPI
Go
Axum
These instructions assume you’ve already set up Tesseral for Express.js.
From there, all of your endpoints can now be called either by User access tokens or by API Keys.
You can detect whether a request is from an API Key or an Access Token using
credentialsType()
:
These @tesseral/tesseral-express
functions will work the same with either auth
type:
However,
accessTokenClaims()
will throw a NotAnAccessTokenError
for requests that are from an API Key
instead of an access token. You can either try / catch
for these errors, do an
credentialsType
check before calling accessTokenClaims()
.